bno085 在Linux使用python的串口 读取
安装
adafruit_bno08x
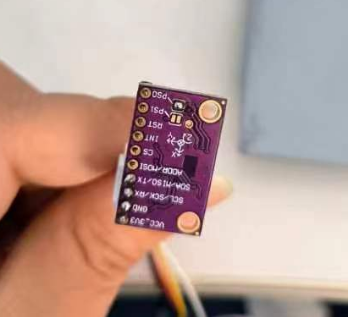
pip3 install adafruit-circuitpython-bno08x
pip3 install adafruit-circuitpython-bno08x-rvc
read_bno.py
import adafruit_bno08x
from adafruit_bno08x_rvc import BNO08x_RVC
import serial
import time
import numpy as np
import argparse
import os
import math
import time
import sys
import datetime
from paho.mqtt import client as mqtt_client
import random
import threading
import json
uart = serial.Serial("/dev/ttyS3", 115200)
#MQTT相关
broker = '127.0.0.1'
#broker = 'www.woyilian.com'
port = 1883
topic = "/sensors/bno085"
cmd_topic = "/cmd/vel"
client_id = 'python-mqtt-{}'.format(random.randint(0, 1000))
#定义mqtt的client
client = mqtt_client.Client(client_id)
rvc = BNO08x_RVC(uart)
def getcmd(roll,pitch,yaw,ax,ay,az):
data = {"roll":"%2.2f"%(roll),"pitch":"%2.2f"%(pitch),"yaw":"%2.2f"%(yaw),"ax":"%f" %(ax),"ay":"%f" %(ay),"az":"%f" %(az)
}
return data
def read_bno085():
time.sleep(0.1)
while True:
yaw, pitch, roll, x_accel, y_accel, z_accel = rvc.heading
print("Yaw: %2.2f Pitch: %2.2f Roll: %2.2f Degrees" % (yaw, pitch, roll))
print("Acceleration X: %2.2f Y: %2.2f Z: %2.2f m/s^2" % (x_accel, y_accel, z_accel))
print("")
cmd =getcmd(roll,pitch,yaw,x_accel,y_accel,z_accel)
json_data = json.dumps(cmd)
result = client.publish(topic,json_data,0)
# result: [0, 1]
status = result[0]
if status == 0:
print(f"Send to topic `{topic}`")
else:
print(f"Failed to send message to topic {topic}")
th1 = threading.Thread(target = read_bno085)
th1.setDaemon(True)
th1.start()
def on_connect(client, userdata, flags, rc):
print("Connected with result code "+str(rc))
def on_message(client, userdata, msg):
global _switch
#print(msg.topic+" "+msg.payload.decode("utf-8"))
_switch = ord(msg.payload.decode("utf-8"))-48
print("%d"%_switch)
def publish(client):
global last_time
global detect_ok
global resultImg
msg_count = 0
while True:
time.sleep(0.001)
if __name__ == '__main__':
print(" connected!")
client.on_connect = on_connect
client.on_message = on_message
client.connect(broker, port, 60)
#publish(client)
# 订阅主题
#client.subscribe(cmd_topic)
client.loop_forever()